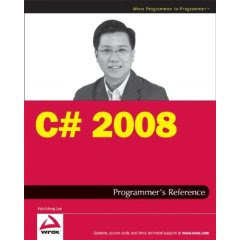
I am extremely excited to inform that I have finished writing my latest book -
C# 2008 Programmer's Reference (available in Nov 08). This is by far the largest book that I have written - close to 800 pages and containing 20 chapters and three appendices. It covers the C# language and also the different types of cool applications you can write using it - Windows app, ASP.NET apps,
Silverlight, Windows Mobile apps, and
WCF.
This book is ideally suited to be used as a course book for a semester course on C# programming and would be useful to students as well as
practitioners embarking on C# programming. Hence, if you are a professor in an institution teaching a course in C# programming and would like to review this book for possible adoption, please send me a message at
weimenglee@learn2develop.net and I would be happy to send you a review copy. For your convenience, I have provided the tables of contents for the book below:
Part 1 – Language Fundamentals
* Chapter 1 introduces the .NET Framework. You will gain an understanding of the key components in the .NET Framework as well as the role played by each of the components. In addition, you will also learn about the relationships between the various versions of the framework, from version 1.0 to the latest 3.5.
* Chapter 2 covers the use of Microsoft Visual Studio 2008 as the tool for C# development. Visual Studio 2008 is an extremely versatile and powerful environment for developing .NET applications. This chapter explores some of the commonly used features that you will likely use in the process of your development work.
* Chapter 3 introduces the syntax of the C# language and covers all the important topics - C# keywords, variables, constants, comments, XML documentation, data types, flow control, loops,
operators and
preprocessor directives.
* Chapter 4 covers one of the most important topics in C# programming—classes and objects. Classes are essentially templates in from which you create objects from. In C# .NET programming, everything you deal with involves classes and objects. This chapter will give you a firm foundation in the use and creation of classes for code reuse.
* Chapter 5 discusses how interfaces can be used to define the contract for a class. You will learn the difference between an interface and an abstract class.
* Chapter 6 looks at how inheritance facilitates code reuse and allows you to extend the functionality of code that you have already written. After this chapter, you will learn about the different types of inheritance and how to define overloaded methods and operators.
* Chapter 7 introduces the concept of delegates and events used in object oriented programming. You will learn what a delegate is and how delegates are used to implement events.
* Chapter 8 examines strings handling in C# and the various ways to manipulate them. For more complex strings pattern matching, you can use regular expressions. This chapter also covers the various ways to format your strings data.
* Chapter 9 discusses the basics of generics and how you can use it to enhance efficiency and type-safety in your applications. Generics enable developers to define type-safe data structures without binding to specific fixed data types at design time.
* Chapter 10 explains how to write
multithreaded applications using the Thread class in the .NET Framework. You will learn how to create and synchronize threads as well as how to write thread-safe Windows applications.
* Chapter 11 examines the concepts of files and streams in .NET. With streams, you can perform a wide range of tasks including compressing and decompressing data, serializing and
de-serializing data, and encrypting and decrypting data. This chapter covers the various ways to manipulate files and the various stream objects in .NET.
* Chapter 12 covers exception handling. An exception is a situation that occurs when your program encounters an error that it is not expecting during
runtime. Understand how to handle exceptions will make your program more robust and resilient.
* Chapter 13 examines arrays and collections. It discusses the many collection classes that you can use to represent groups of data in .NET.
* Chapter 14 introduces the new feature in .NET 3.5 – Language Integrated Query (
LINQ). It covers all the important implementations of
LINQ –
LINQ to Objects,
LINQ to XML,
LINQ to
Dataset, and
LINQ to
SQL.
* Chapter 15 explores the concept of assemblies. In .NET, the basic unit
deployable is called an assembly. Assemblies play an important part of the development process where understanding how they work is useful in helping you develop scalable and efficient .NET applications.
Part 2 – Applications Development using C#
* Chapter 16 demonstrates how you can build a Windows application using the C# language. The sample application demonstrated shows how to perform FTP using the classes available in the .NET Framework. You will also learn how to perform printing in a.NET application and how to deploy Windows applications using the
ClickOnce technology.
* Chapter 17 demonstrates how you can use C# to build an ASP.NET web application. You will learn to perform data-binding using the new
LinqDataSource control and how to AJAX-enable your web pages.
* Chapter 18 illustrates Windows Mobile development using the .NET Compact Framework, a subset of the .NET Framework. You will learn the basics of the Windows Mobile development and build a sample
RSS reader application. Finally, you will create a professional setup package for your application so that it can be distributed to your readers for installation.
* Chapter 19 helps you get started with
Silverlight, and provides an opportunity for you to get a feel for how
Silverlight development works. It covers
Silverlight 1.0 and 2, and contains several examples showing the capabilities of
Silverlight – animation, media, .NET integration, etc.
* Chapter 20 provides a quick introduction to the new Windows Communication Foundation (
WCF) technology and shows how it addresses some of the limitations of today’s web services technology. While most books and conference focused heavily on the theory behind
WCF, this chapter shows you how to build
WCF services and then explains the theory behind them. It ends with an example that shows how to build a ticketing application that allows multiple clients to obtain updated
seta information real-time.
Part 3 – Appendices
* Appendix A lists the various keywords in C# that are predefined and have special meanings to the compiler.
* Appendix B summarizes the features of the various versions of the .NET Framework and how to use the Object Browser feature in Visual Studio 2008 to browse the available
namespaces and classes in the .NET Framework.
* Appendix C shows you how to generate
MSDN-style documentation for your project using Visual Studio 2008 and a third-party documentation generation tool—Sandcastle.