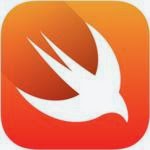
class MySingleton {
//---typed property to return a shared instance of the current class---
class var sharedInstance: MySingleton {
struct Static {
//---to contain the one and only instance of this class---
static var instance: MySingleton!
//---a token to ensure that the class is only instantiated once---
static var token: dispatch_once_t = 0
}
//---executes a block object once and only once for the lifetime of an application---
dispatch_once(&Static.token) {
//---creates an instance of this class---
Static.instance = MySingleton()
}
//---returns the instance of this class---
return Static.instance
}
//---the properties in your class---
var num:Int = 0
var str:String = ""
}
In the above code snippet, MySingleton is a singleton, i.e. you can only create one and only one instance of this class. To make use of this singleton, use the following statements:
var s1 = MySingleton.sharedInstance
s1.num = 5
s1.str = "Hello Singleton!"
println(s1.num) //---5---
println(s1.str) //---Hello Singleton!---
var s2 = MySingleton.sharedInstance
println(s2.num) //---5---
println(s2.str) //---Hello Singleton!---
Have fun with Swift!
No comments:
Post a Comment