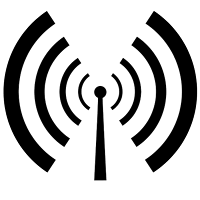
The easiest way to check if the connectivity option available on
your iOS device is to use the Reachability
class provided by Apple. To do so, download the code sample from: https://developer.apple.com/library/ios/samplecode/reachability/Introduction/Intro.html
and copy the Reachability.h and Reachability.m file into your own project.
Next, add a reference to the SystemConfiguration.framework
in your project.
To use the Reachability
class, import its header file:
#import "Reachability.h"
Finally, use the following code snippet to check the type of
connectivity you have on your iOS device:
Reachability
*reachability =
[Reachability
reachabilityForInternetConnection];
[reachability
startNotifier];
NetworkStatus
networkStatus =
[reachability
currentReachabilityStatus];
if(networkStatus ==
NotReachable) {
NSLog(@"No
Internet");
}
else if (networkStatus
== ReachableViaWiFi) {
NSLog(@"Wi-Fi");
}
else if (networkStatus
== ReachableViaWWAN) {
NSLog(@"3G");
}
[reachability
stopNotifier];
No comments:
Post a Comment